Notiz
Klicken Sie hier , um den vollständigen Beispielcode herunterzuladen
Fadenkreuz-Cursor #
Dieses Beispiel fügt ein Fadenkreuz als Datencursor hinzu. Das Fadenkreuz ist als reguläres Linienobjekt implementiert, das bei Mausbewegung aktualisiert wird.
Wir zeigen drei Implementierungen:
Eine einfache Cursor-Implementierung, die die Figur bei jeder Mausbewegung neu zeichnet. Dies ist etwas langsam und Sie bemerken möglicherweise eine Verzögerung der Fadenkreuzbewegung.
Ein Cursor, der Blitting zur Beschleunigung des Renderings verwendet.
Ein Cursor, der an Datenpunkten einrastet.
Ein schnelleres Bewegen des Cursors ist mit nativem GUI-Zeichnen möglich, wie in Hinzufügen eines Cursors in WX .
Die Drittanbieterpakete mpldatacursor und mplcursors können verwendet werden, um einen ähnlichen Effekt zu erzielen.
import matplotlib.pyplot as plt
import numpy as np
class Cursor:
"""
A cross hair cursor.
"""
def __init__(self, ax):
self.ax = ax
self.horizontal_line = ax.axhline(color='k', lw=0.8, ls='--')
self.vertical_line = ax.axvline(color='k', lw=0.8, ls='--')
# text location in axes coordinates
self.text = ax.text(0.72, 0.9, '', transform=ax.transAxes)
def set_cross_hair_visible(self, visible):
need_redraw = self.horizontal_line.get_visible() != visible
self.horizontal_line.set_visible(visible)
self.vertical_line.set_visible(visible)
self.text.set_visible(visible)
return need_redraw
def on_mouse_move(self, event):
if not event.inaxes:
need_redraw = self.set_cross_hair_visible(False)
if need_redraw:
self.ax.figure.canvas.draw()
else:
self.set_cross_hair_visible(True)
x, y = event.xdata, event.ydata
# update the line positions
self.horizontal_line.set_ydata(y)
self.vertical_line.set_xdata(x)
self.text.set_text('x=%1.2f, y=%1.2f' % (x, y))
self.ax.figure.canvas.draw()
x = np.arange(0, 1, 0.01)
y = np.sin(2 * 2 * np.pi * x)
fig, ax = plt.subplots()
ax.set_title('Simple cursor')
ax.plot(x, y, 'o')
cursor = Cursor(ax)
fig.canvas.mpl_connect('motion_notify_event', cursor.on_mouse_move)
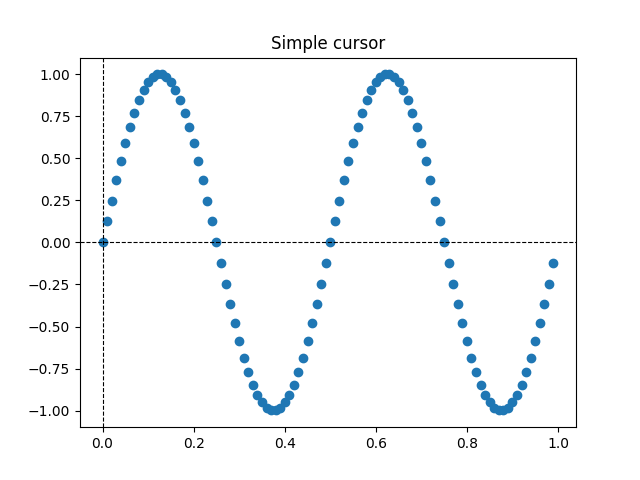
11
Schnelleres Neuzeichnen mit Blitting #
Diese Technik speichert den gerenderten Plot als Hintergrundbild. Nur die geänderten Künstler (Fadenkreuze und Text) werden neu gerendert. Sie werden durch Blitting mit dem Hintergrund kombiniert.
Diese Technik ist deutlich schneller. Es erfordert etwas mehr Einrichtung, da der Hintergrund ohne die Fadenkreuze gespeichert werden muss (siehe
create_new_background()
). Außerdem muss bei jeder Änderung der Figur ein neuer Hintergrund erstellt werden. Dies wird durch die Verbindung mit dem erreicht
'draw_event'
.
class BlittedCursor:
"""
A cross hair cursor using blitting for faster redraw.
"""
def __init__(self, ax):
self.ax = ax
self.background = None
self.horizontal_line = ax.axhline(color='k', lw=0.8, ls='--')
self.vertical_line = ax.axvline(color='k', lw=0.8, ls='--')
# text location in axes coordinates
self.text = ax.text(0.72, 0.9, '', transform=ax.transAxes)
self._creating_background = False
ax.figure.canvas.mpl_connect('draw_event', self.on_draw)
def on_draw(self, event):
self.create_new_background()
def set_cross_hair_visible(self, visible):
need_redraw = self.horizontal_line.get_visible() != visible
self.horizontal_line.set_visible(visible)
self.vertical_line.set_visible(visible)
self.text.set_visible(visible)
return need_redraw
def create_new_background(self):
if self._creating_background:
# discard calls triggered from within this function
return
self._creating_background = True
self.set_cross_hair_visible(False)
self.ax.figure.canvas.draw()
self.background = self.ax.figure.canvas.copy_from_bbox(self.ax.bbox)
self.set_cross_hair_visible(True)
self._creating_background = False
def on_mouse_move(self, event):
if self.background is None:
self.create_new_background()
if not event.inaxes:
need_redraw = self.set_cross_hair_visible(False)
if need_redraw:
self.ax.figure.canvas.restore_region(self.background)
self.ax.figure.canvas.blit(self.ax.bbox)
else:
self.set_cross_hair_visible(True)
# update the line positions
x, y = event.xdata, event.ydata
self.horizontal_line.set_ydata(y)
self.vertical_line.set_xdata(x)
self.text.set_text('x=%1.2f, y=%1.2f' % (x, y))
self.ax.figure.canvas.restore_region(self.background)
self.ax.draw_artist(self.horizontal_line)
self.ax.draw_artist(self.vertical_line)
self.ax.draw_artist(self.text)
self.ax.figure.canvas.blit(self.ax.bbox)
x = np.arange(0, 1, 0.01)
y = np.sin(2 * 2 * np.pi * x)
fig, ax = plt.subplots()
ax.set_title('Blitted cursor')
ax.plot(x, y, 'o')
blitted_cursor = BlittedCursor(ax)
fig.canvas.mpl_connect('motion_notify_event', blitted_cursor.on_mouse_move)
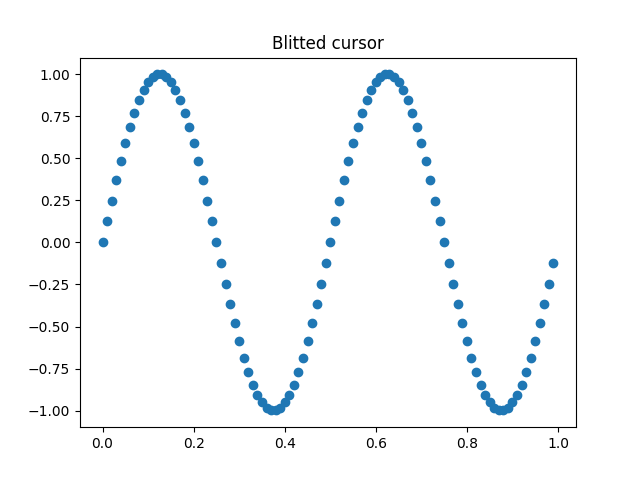
12
Fangen an Datenpunkten #
Der folgende Cursor rastet an den Datenpunkten eines Line2D
Objekts ein.
Um unnötiges Neuzeichnen zu vermeiden, wird der Index des zuletzt angezeigten Datenpunkts in gespeichert self._last_index
. Erst wenn sich die Maus so weit bewegt, dass ein weiterer Datenpunkt ausgewählt werden muss, wird ein Neuzeichnen ausgelöst. Dies reduziert die Verzögerung aufgrund vieler Neuzeichnungen. Natürlich könnte für zusätzliche Beschleunigung noch Blitting hinzugefügt werden.
class SnappingCursor:
"""
A cross hair cursor that snaps to the data point of a line, which is
closest to the *x* position of the cursor.
For simplicity, this assumes that *x* values of the data are sorted.
"""
def __init__(self, ax, line):
self.ax = ax
self.horizontal_line = ax.axhline(color='k', lw=0.8, ls='--')
self.vertical_line = ax.axvline(color='k', lw=0.8, ls='--')
self.x, self.y = line.get_data()
self._last_index = None
# text location in axes coords
self.text = ax.text(0.72, 0.9, '', transform=ax.transAxes)
def set_cross_hair_visible(self, visible):
need_redraw = self.horizontal_line.get_visible() != visible
self.horizontal_line.set_visible(visible)
self.vertical_line.set_visible(visible)
self.text.set_visible(visible)
return need_redraw
def on_mouse_move(self, event):
if not event.inaxes:
self._last_index = None
need_redraw = self.set_cross_hair_visible(False)
if need_redraw:
self.ax.figure.canvas.draw()
else:
self.set_cross_hair_visible(True)
x, y = event.xdata, event.ydata
index = min(np.searchsorted(self.x, x), len(self.x) - 1)
if index == self._last_index:
return # still on the same data point. Nothing to do.
self._last_index = index
x = self.x[index]
y = self.y[index]
# update the line positions
self.horizontal_line.set_ydata(y)
self.vertical_line.set_xdata(x)
self.text.set_text('x=%1.2f, y=%1.2f' % (x, y))
self.ax.figure.canvas.draw()
x = np.arange(0, 1, 0.01)
y = np.sin(2 * 2 * np.pi * x)
fig, ax = plt.subplots()
ax.set_title('Snapping cursor')
line, = ax.plot(x, y, 'o')
snap_cursor = SnappingCursor(ax, line)
fig.canvas.mpl_connect('motion_notify_event', snap_cursor.on_mouse_move)
plt.show()
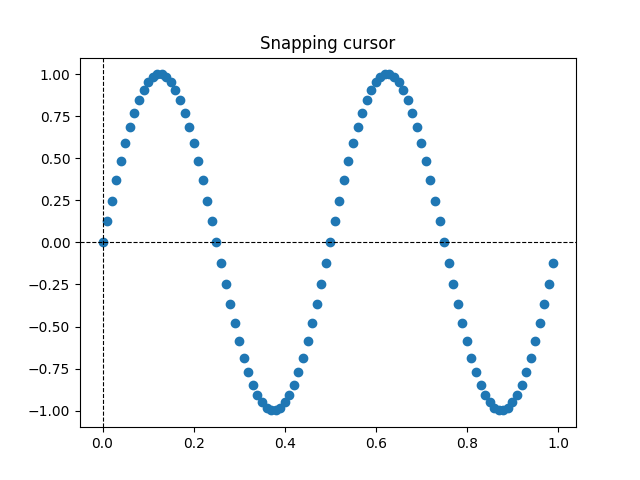
Gesamtlaufzeit des Skripts: ( 0 Minuten 1.133 Sekunden)