Notiz
Klicken Sie hier , um den vollständigen Beispielcode herunterzuladen
Benutzerdefinierte Boxstile #
Dieses Beispiel zeigt die Implementierung einer benutzerdefinierten BoxStyle
. Benutzerdefinierte ConnectionStyle
s und ArrowStyle
s können auf ähnliche Weise definiert werden.
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
Benutzerdefinierte Rahmenstile können als Funktion implementiert werden, die Argumente annimmt, die sowohl einen rechteckigen Rahmen als auch die Menge der "Mutation" angeben, und den "mutierten" Pfad zurückgibt. Die spezifische Signatur ist die
custom_box_style
untenstehende.
Hier geben wir einen neuen Pfad zurück, der links vom Feld eine "Pfeil" -Form hinzufügt.
Der benutzerdefinierte Boxstil kann dann verwendet werden, indem Sie
an übergeben .bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
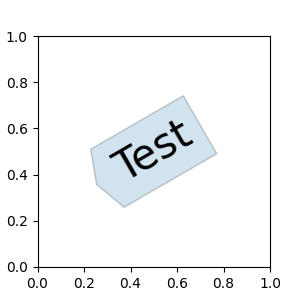
Text(0.5, 0.5, 'Test')
Ebenso können benutzerdefinierte Boxstile als Klassen implementiert werden, die
__call__
.
Die Klassen können dann im Diktat registriert werden BoxStyle._style_list
, wodurch der Boxstil als Zeichenfolge angegeben werden kann
. Beachten Sie, dass diese Registrierung auf internen APIs basiert und daher nicht offiziell unterstützt wird.bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
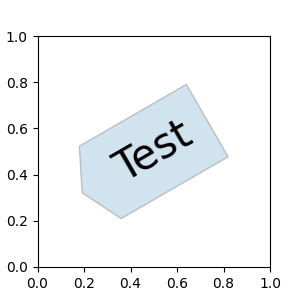