Notiz
Klicken Sie hier , um den vollständigen Beispielcode herunterzuladen
Erstellen von Histogrammen mit Rectangles und PolyCollections #
Verwenden eines Pfadpatches zum Zeichnen von Rechtecken. Die Technik der Verwendung vieler Rectangle-Instanzen oder die schnellere Methode der Verwendung von PolyCollections wurden implementiert, bevor wir die richtigen Pfade mit moveto/lineto, closepoly usw. in mpl hatten. Jetzt, da wir sie haben, können wir Sammlungen von regelmäßig geformten Objekten mit homogenen Eigenschaften effizienter mit einer PathCollection zeichnen. Dieses Beispiel erstellt ein Histogramm – es ist arbeitsintensiver, die Vertex-Arrays am Anfang einzurichten, aber es sollte viel schneller für eine große Anzahl von Objekten sein.
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.path as path
fig, ax = plt.subplots()
# Fixing random state for reproducibility
np.random.seed(19680801)
# histogram our data with numpy
data = np.random.randn(1000)
n, bins = np.histogram(data, 50)
# get the corners of the rectangles for the histogram
left = bins[:-1]
right = bins[1:]
bottom = np.zeros(len(left))
top = bottom + n
# we need a (numrects x numsides x 2) numpy array for the path helper
# function to build a compound path
XY = np.array([[left, left, right, right], [bottom, top, top, bottom]]).T
# get the Path object
barpath = path.Path.make_compound_path_from_polys(XY)
# make a patch out of it
patch = patches.PathPatch(barpath)
ax.add_patch(patch)
# update the view limits
ax.set_xlim(left[0], right[-1])
ax.set_ylim(bottom.min(), top.max())
plt.show()
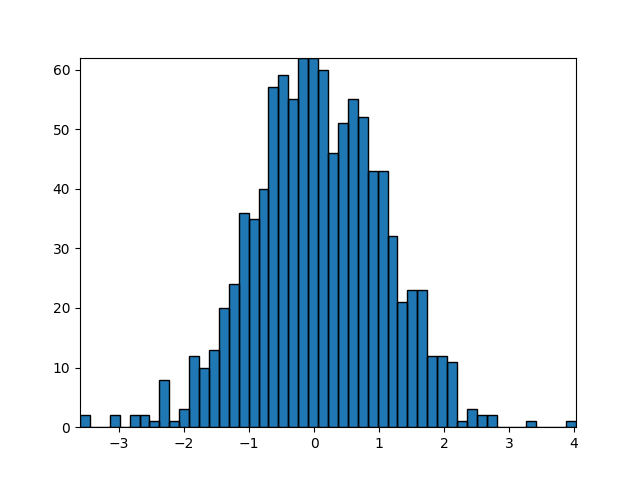
Es sollte beachtet werden, dass wir, anstatt ein dreidimensionales Array zu erstellen und zu verwenden make_compound_path_from_polys
, den zusammengesetzten Pfad auch direkt erstellen könnten, indem wir Scheitelpunkte und Codes verwenden, wie unten gezeigt
nrects = len(left)
nverts = nrects*(1+3+1)
verts = np.zeros((nverts, 2))
codes = np.ones(nverts, int) * path.Path.LINETO
codes[0::5] = path.Path.MOVETO
codes[4::5] = path.Path.CLOSEPOLY
verts[0::5, 0] = left
verts[0::5, 1] = bottom
verts[1::5, 0] = left
verts[1::5, 1] = top
verts[2::5, 0] = right
verts[2::5, 1] = top
verts[3::5, 0] = right
verts[3::5, 1] = bottom
barpath = path.Path(verts, codes)
Verweise
In diesem Beispiel wird die Verwendung der folgenden Funktionen, Methoden, Klassen und Module gezeigt:
Dieses Beispiel zeigt eine Alternative zu